1. Overview
In this short article, we’ll go over a couple of ways to comment and uncomment lines in vi and vim.
2. Setup
Let’s say we’re using vim to edit a small shell script:
#!/bin/bash
echo 'Hello, World!'
echo 'Here is a script to do some math!'
echo 3 + 2 = $((3+2))
If we execute this script, we’ll see the following:
$ ./script.sh
Hello, World!
Here is a script to do some math!
3 + 2 = 5
Let’s say we want to comment out the last two lines. There are several quick ways to do this using vi or vim.
3. Visual Block Mode
vim has an editing mode called Visual Block Mode. In this mode, we can edit a rectangular selection of text. Let’s try it out.
3.1. Adding Comments
To start, let’s move the cursor to the beginning of line 4 in our script using the key bindings (any other vim navigation would work also):
4 G
We enter visual block mode by using the following:
CTRL+v
Then, we can use j to add the fifth line of the script to our selection. Our vim terminal should look like this:
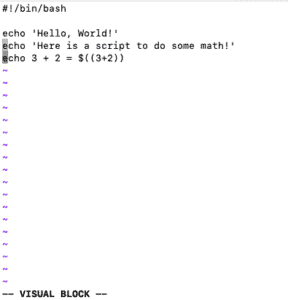
Once we have our lines selected, we’ll add a comment. Since this is a Bash script, the comment will be a #. To enter Insert mode, we use this binding:
SHIFT+I
Typing the comment character and a space after, our terminal should now look like this:
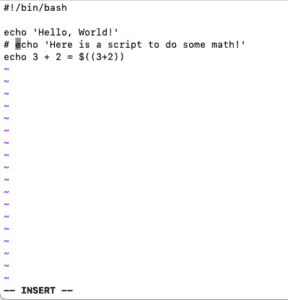
Finally, we can hit Esc to apply that change to the lines selected earlier. The change we did on one line will now apply to the full selection, and our script is now:
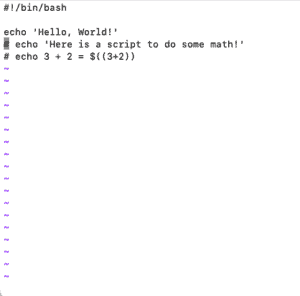
3.2. Toggling Comments
Once we’ve added comments, we can toggle the comments added in visual block mode by using u to undo them and CTRL+r to redo them. Note this won’t work if we make other edits to the file.
3.3. Removing Comments
Similar to adding comments in visual block mode, we can similarly remove them. Currently, our script is:
#!/bin/bash
echo 'Hello, World!'
# echo 'Here is a script to do some math!'
# echo 3 + 2 = $((3+2))
Let’s navigate to line 4 again. Now, we’ll enter visual block mode using CTRL+v. Then, hit j to add line 5 to our selection. Since we want to remove the # and the extra space, let’s increase our rectangular selection to include the second column by using l (lowercase L). Now our terminal looks like:
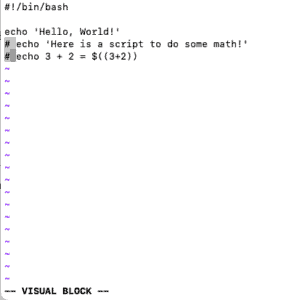
Finally, we’ll hit x to delete everything in the rectangular selection and also exit visual block mode. Now we are back to where we were at the beginning:
#!/bin/bash
echo 'Hello, World!'
echo 'Here is a script to do some math!'
echo 3 + 2 = $((3+2))
4. Commenting Using Substitutions
Another way we can add and remove comments in vim is by using search and replace.
4.1. Normal Mode
Let’s start again with our test script:
#!/bin/bash
echo 'Hello, World!'
echo 'Here is a script to do some math!'
echo 3 + 2 = $((3+2))
Now, let’s say we want to comment out lines 4 and 5. First, we enter command-line mode by typing : (colon). Now, we’ll specify a range and the command we want to use to comment:
:4,5s/^/#
Breaking down this command, we have:
- the numbers are the range of lines
- s means “substitute”
- ^ is the beginning of the line
- # is the character we are inserting
Hitting Enter, we’ll now see the lines commented out:
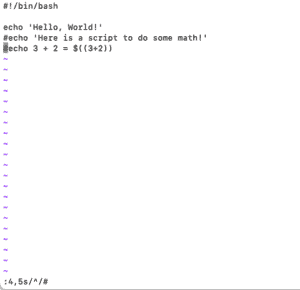
To undo the comments, we can switch the search and replace statement to:
:4,5s/^#/
This replaces any # at the beginning of lines within the range with nothing, as there is nothing after the second slash in the command:
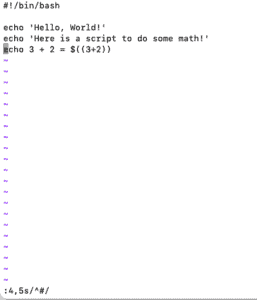
4.2. Visual Mode
As a slight variation on the substitution above, we can use visual mode to select the lines to comment. Let’s first move to line 4 by typing 4G and then v to enter visual mode and j to expand the selection down one line. Our session should look like this now:
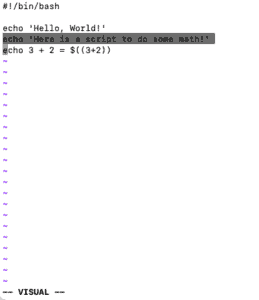
And now, we can search and replace this selection. Enter command-line mode by typing :. Notice that since we are entering command-line mode from visual mode, vim pre-populates the prompt with the selection range:
'<,'>
This will apply whatever command comes after to just the selected area. We’ll add our search and replace:
s/^/#
We can then hit Enter to comment our lines:
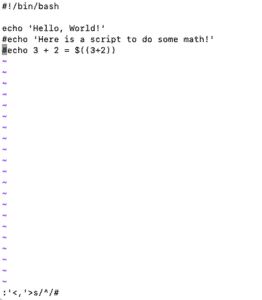
To undo the comments, we can do the reverse search and replace:
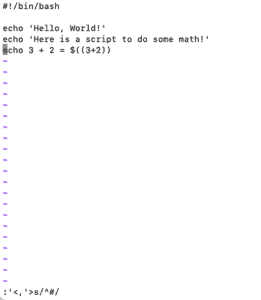
5. Plugins
While beyond the scope of this article, there are plugins like The NERD Commenter that we could install to allow for easy commenting on many different file types.
6. Conclusion
In this article, we presented a few different ways to comment and uncomment lines in vim. We looked at how to add and remove comments using inserts in visual block mode. We also looked out using search and replace commands.