1. Overview
Capturing user input from dialog boxes is a common task when working with Bash scripts. Whether we’re creating interactive command-line utilities or automating tasks, understanding how to handle dialog box input efficiently is essential.
In this article, we’ll explore two distinct methods for achieving this goal. We’ll start with the traditional approach of redirecting the output to a temporary file. Next, we’ll introduce a more concise method using the –output-fd flag in the dialog utility. Lastly, we’ll compare the two methods side by side, considering simplicity, readability, and efficiency.
2. Capturing Dialog Box Input
In Bash scripting, retrieving and storing user input from a dialog box presents unique challenges compared to standard input methods. Dialog boxes, commonly used for interactive interfaces in shell scripts, often require special handling to direct their input into variables for further processing.
2.1. Challenges of Direct Capture
The major obstacle here is that dialog boxes typically output their content to the standard error stream, stderr, instead of the standard output stream, stdout. Consequently, traditional methods like command substitution cannot directly capture dialog box input.
Let’s consider this line of code:
$ user_input=$(dialog --title "Create Directory" --inputbox "Enter the directory name:" 8 40)
In theory, this should store the user’s input into the user_input variable. However, when we run this, we’ll notice that the command line appears to hang indefinitely, waiting for input.
This happens because when we use command substitution $(…), it captures the command’s stdout into the variable. In the case of dialog, since the user input is on stderr, it doesn’t get captured as expected. Interestingly, if we type something (even though it’s not visible), it’ll be written to stderr and still appear on the screen. This happens because stderr still points to the current terminal.
As a result, we need to employ alternative methods, such as redirection or temporary file handling, to effectively capture the input. These methods involve redirecting the output of the dialog box to a file or stream, and then reading the contents of that file or stream into a variable for further processing.
2.2. Importance of Storing Input in Variables
Storing dialog box input in variables is crucial for several reasons. Firstly, it allows for easy manipulation and processing of user-provided data within the script. Additionally, variables provide a convenient way to pass user input to other parts of the script or external commands for further processing. Furthermore, storing input in variables facilitates error checking and validation, enabling scripts to handle user responses effectively.
3. Redirection With Temporary Stream
Redirection with a temporary stream involves temporarily redirecting the standard error to capture the user input in a variable. This method employs file descriptors to redirect the dialog box input to a temporary location, allowing us to capture it in a variable:
$ cat redirect_with_temp_stream.sh
#!/bin/bash
# Redirecting dialog box output to a temporary stream
exec 3>&1 # Save the place that stdout (1) points to
result=$(dialog --title "Input Required" --inputbox "Enter your name:" 10 30 2>&1 >/dev/tty)
exec 3>&- # Close the temporary stream
echo "Hello, $result"
Let’s understand what’s happening here:
- We start by opening a temporary stream using exec 3>&1. This creates a new file descriptor 3 that points to the same location as the standard output file descriptor.
- The dialog command displays an input box for the user. The 2>&1 redirection ensures that both regular output stdout and error messages stderr are sent to the same location, file descriptor 1.
- Since we’re redirecting both stdout and stderr, we’ll need >/dev/tty redirection to display the dialog box on the terminal screen.
- After capturing the input, we close the temporary stream using exec 3>&-
- Finally, we echo a personalized greeting using the value stored in the result variable.
Let’s give it a try:
$ bash redirect_with_temp_stream.sh
First, the dialog box is displayed, and we can type in a name:
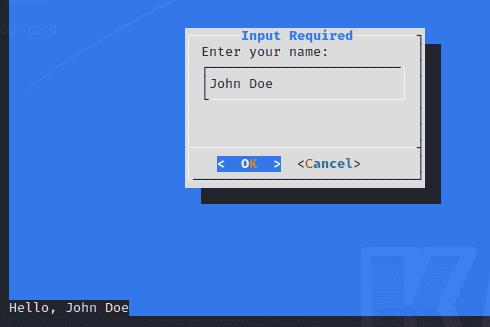
The user input is successfully stored in the result variable.
4. Using the –output-fd Flag
The –output-fd flag in the dialog utility enables us to specify the file descriptor fd to which the output, including user input, should be directed. File descriptors are numeric identifiers used by the operating system to manage input and output streams. By using this flag, we can direct the output of the dialog box, which typically goes to the stderr stream, to a specific file descriptor of our choice.
Thus, we can capture the dialog box input directly into variables without resorting to intermediate files or complex redirection:
$ cat output-fd-flag.sh
#!/bin/bash
# Capture user input using dialog
result=$(dialog --output-fd 1 --title "Input Required" --inputbox "Enter your name:" 10 30)
# Echo the user input
echo "Hello $result"
In this case, the –output-fd 1 flag explicitly sets the output to stdout (file descriptor 1).
Again, the dialog box pops up when we run the script:
Similarly, we successfully direct the dialog box input to the result variable.
This approach simplifies the process of capturing user input, eliminating the need for complex redirection or temporary file handling. It also offers flexibility in directing output to different file descriptors, allowing us to tailor the behavior of our scripts based on specific requirements or preferences.
Additionally, it facilitates integration with other command-line utilities and scripting tools that utilize file descriptors for input and output redirection.
5. Comparison of Both Methods
We’ve discussed two methods of directing dialog box input to a variable, namely redirection with a temporary stream and using the –output-fd flag. Let’s compare them based on ease of implementation, efficiency, and flexibility:
Comparison Factor | Redirection with Temporary Stream | Using –output-fd Flag |
---|---|---|
Ease of Implementation | Requires additional steps to manage file descriptors and handle potential errors | Directly specifies file descriptor without additional steps |
Efficiency | Involves additional file handling and redirection steps, potentially impacting performance | Streamlines the code by eliminating intermediate steps, improving efficiency |
Flexibility | Provides flexibility in directing output to different file descriptors | Offers flexibility in specifying the file descriptor for output, allowing customization |
In summary, while both methods offer ways to capture dialog box input into variables, the choice between them depends on the specific requirements of the script and the desired level of flexibility and efficiency.
6. Conclusion
In this article, we explored two primary methods for directing the dialog box input to a variable, redirection with a temporary stream and using the –output-fd flag with the dialog utility. Each method has its advantages and considerations. Redirection with a temporary stream offers flexibility in directing output to different file descriptors, allowing for customized behavior. However, it involves additional file handling and redirection steps, which may impact performance.
On the other hand, using the –output-fd flag streamlines the code by eliminating intermediate steps, improving efficiency. While it may be more straightforward to implement, it provides less flexibility compared to redirection with a temporary stream.
Ultimately, the choice between these methods depends on the specific requirements of the script and the desired balance between flexibility and efficiency. By understanding the strengths and limitations of each approach, we can make informed decisions to effectively capture dialog box input and enhance the interactivity of our Bash scripts.