1. Introduction
A connection timeout error occurs when a client application attempts to connect with a server, but the server takes too long to respond. This can happen due to network congestion, server overload, or misconfigured settings.
In this tutorial, we’ll explore some techniques to artificially create a connection timeout error.
2. Reasons to Simulate the Connection Timeout Error
Introducing connection timeouts allows us to test how well our application handles network-related issues:
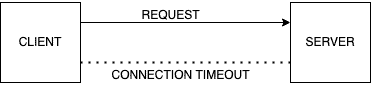
With it, we can test if the application falls back to alternative mechanisms or provides users with appropriate feedback when a connection cannot be established within the expected time.
Additionally, it allows us to assess how our application scales under different network load levels and whether it can handle simultaneous connection attempts without experiencing excessive timeouts.
3. Connecting to a Non-Routable IP Address
Private networks reserve non-routable IP addresses, also known as private IP addresses, for internal use. These addresses aren’t intended to be routed over the public internet. A router with network address translation (NAT) capability is used to translate between private and public IP addresses, enabling devices within a private network to access resources on the internet. Therefore, we can implement the following ways to prevent this translation and cause the timeout error.
3.1. Configure a Static Route
When configuring a static route, we provide explicit instructions to the router about how to reach a particular network or device. This overrides the router’s normal routing behavior.
So, we can configure a static route to lead to an unreachable destination. As a result, the router cannot establish a connection, leading to an eventual timeout error.
3.2. Disable NAT for the Device
Disabling a device’s Network Address Translation (NAT) can lead to connection timeout errors, especially in communication with external networks. If NAT is disabled for a device, it will communicate directly with external servers using its private IP address.
However, external servers expect responses from the public IP address associated with the NAT device. Since the external server receives replies from an unexpected source (the private IP address instead of the NAT device’s public IP address), it may ignore or drop these packets. This mismatch between expected and actual source addresses can lead to connection timeout errors as the external server waits for a response that never arrives.
4. Firewalled Server with Non-standard Port
We can also select a port and configure the firewall to block incoming connections on the chosen non-standard port.
The client’s request will go unanswered since the server’s firewall is configured to block incoming connections on the non-standard port. After a specific duration, a connection timeout error occurs:
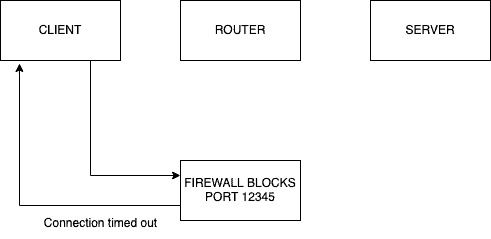
Thus, the firewall actively blocks incoming connections on the non-responsive port, resulting in a connection timeout error at the client’s end.
5. Using a Server on a Non-Responsive Port
We can set up a server on a port where no service or application actively listens. The server doesn’t respond to the incoming connection requests since there’s no active service or application on the selected port. Thus, after sending the connection request, the client waits for a response from the server. However, since the server is not responsive, the client eventually times out:
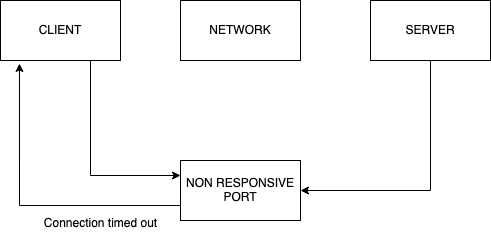
As a result of the timeout, the client generates a connection timeout error.
6. Simulate Timeout Within an Application
We can create a connection timeout error within an application by setting a timeout value for network operations. This ensures that the application will generate a timeout error if it cannot establish a connection within the specified time. Below is an example using Python’s socket module:
def connect_to_server():
# Simulating a connection request
print("Connecting to server...")
# Simulate a non-responsive server by waiting for 10 seconds
time.sleep(10)
# Generate a timeout error
raise TimeoutError("Connection timed out")
In this example, we introduce a 10-second delay using time.sleep() to mimic a non-responsive server. The program raises a TimeoutError if the server doesn’t respond within the allotted time.
7. Software Tools for Simulating the Connection Timeout Error
We can simulate a connection timeout error using software tools like Charles Proxy and Wiremock.
In Charles, we can set up blacklist hosts to create a connection timeout error. Charles provides two ways to simulate the connection timeout: drop the connection or return a 403 status code:
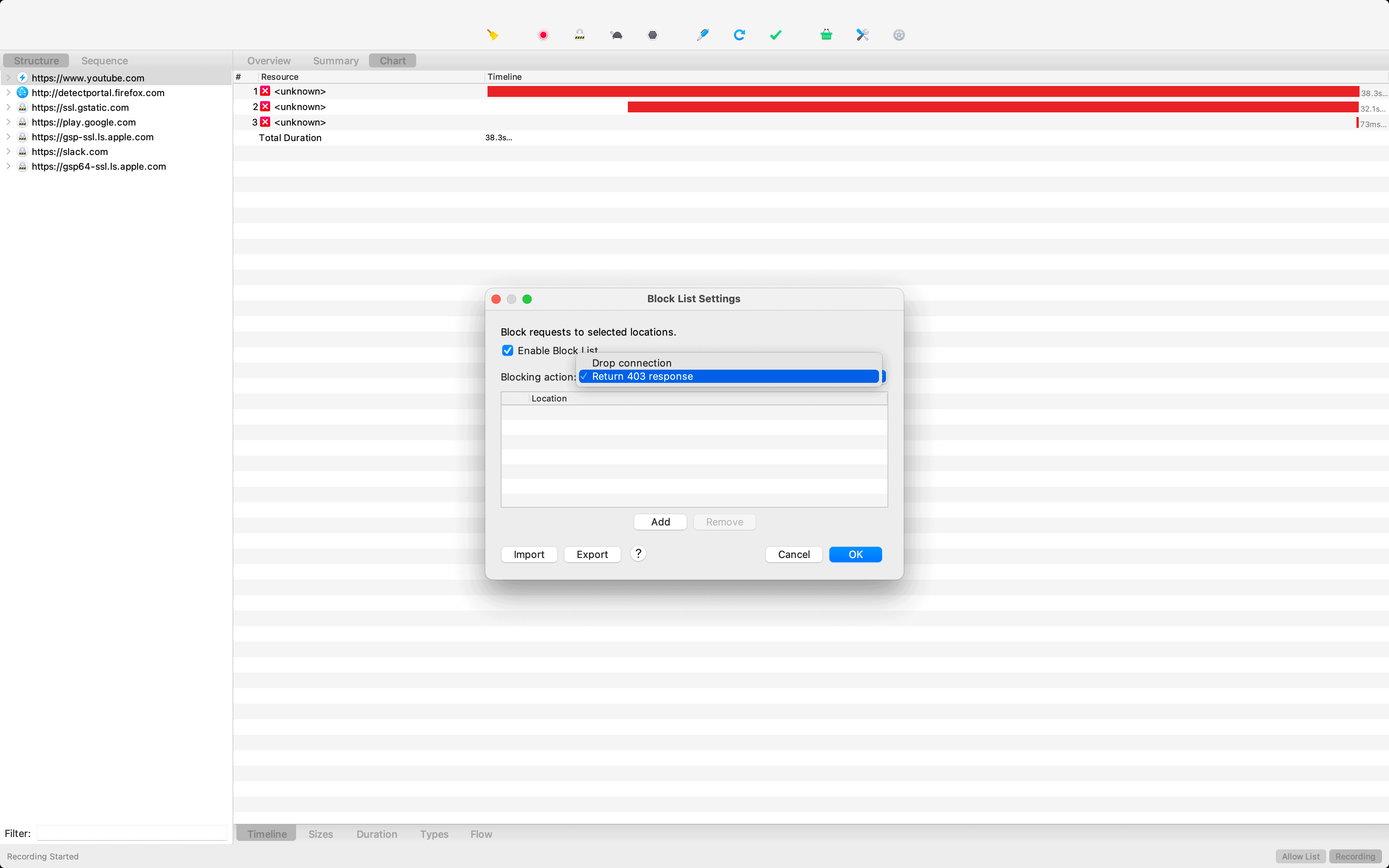
We must ensure that we have SSL Proxying enabled on the API/website to use Charles:
Similarly, Wiremock can also add delays to a network call or create corrupted responses by returning error codes.
8. Conclusion
In this article, we learned about connection timeout errors and various methods of causing them artificially. For example, we can connect to a non-routable IP address using a server hosted on a non-responsive port or simulate a non-responsive server in our code.