1. Introduction
Loop unrolling is an important technique in code optimization, offering a pathway to enhance software performance by strategically restructuring repetitive loops.
At its core, loop unrolling aims to reduce the overhead associated with loop iteration, thereby enabling more efficient execution on modern processors.
In this tutorial, we’ll delve into the essence of loop unrolling, its significance in software development, and its tangible benefits.
2. What is Loop Unrooling?
Loop unrolling involves unwinding a loop by replicating its body multiple times in order to reduce the number of loop iterations required to accomplish a single task. Therefore, instead of executing the loop’s body in its entirety for each iteration, unrolled loops execute multiple iterations within a single loop.
For example, the basic loop with three stages and three iterations can be unrolled loop with three stages and three iterations:
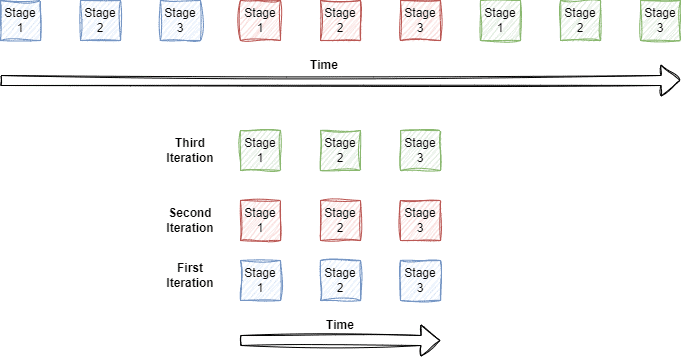
This optimization technique fundamentally alters the program’s control flow, aiming to exploit the underlying hardware more effectively.
By minimizing loop overhead, such as loop control and branching, loop unrolling can lead to significant performance gains, particularly in scenarios where loops constitute a substantial portion of the program’s execution time. Whether optimizing computational algorithms, numerical simulations, or signal processing routines, loop unrolling unlocks latent performance.
3. How Loop Unrolling Works
Instead of executing the loop’s body in its entirety for each iteration, unrolled loops execute multiple iterations within a single loop iteration. This restructuring of the loop alters the control flow of the program, aiming to exploit hardware resources more efficiently.
We can consider a simple loop that iterates over an array, performing a computation on each element:
for i in range(len(array)):
result += array[i]
In its unrolled form, this loop might look like:
for i in range(0, len(array), 2):
result += array[i]
result += array[i+1]
Here, the loop body has been replicated, with each iteration processing two elements of the array instead of one. This reduces the number of loop iterations by half, halving the loop overhead. Depending on our hardware resources, we can reduce even further the loop iterations and improve the overall performance.
By executing multiple iterations within a single loop iteration, loop unrolling amortizes the cost of this overhead, leading to improved performance.
The processor can also exploit parallelism at the instruction level, by executing multiple instructions concurrently and potentially improving throughput.
4. Types of Loop Unrolling
Loop unrolling can be implemented in various ways, each with its own advantages and trade-offs.
4.1. Full Unrolling
In full unrolling, the loop body is replicated entirely, with each iteration of the original loop replaced by multiple iterations of the unrolled loop.
4.2. Partial Unrolling
Partial unrolling involves replicating only a portion of the loop body, typically reducing the number of iterations while still retaining some loop structure.
4.3. Software Pipelining
Software pipelining extends loop unrolling by overlapping the execution of multiple iterations, potentially improving throughput and resource utilization.
5. Benefits of Loop Unrolling
One of the primary benefits of loop unrolling is the reduction in loop overhead. Traditional loops incur overhead due to loop control mechanisms, such as loop condition checks and loop counter increments, which are executed in each iteration.
Furthermore, these methods can also enhance instruction-level parallelism, a key factor in modern processor architectures. Unrolled loops expose more opportunities for instruction-level optimizations, such as instruction pipelining and instruction scheduling. This allows the processor to take more advantage of its available hardware resources.
Memory access patterns can also be improved by processing multiple array elements within a single loop iteration. Therefore, we can result in reduced memory stalls and improved overall performance, particularly in memory-bound applications.
Loop unrolling can also facilitate compiler optimizations, enabling the compiler to generate more efficient code.
Lastly, in cases where loop iterations involve independent and parallelizable computations, such techniques can facilitate the utilization of SIMD instructions, which allow processors to perform the same operation on multiple data elements simultaneously.
6. When to Use Loop Unrolling
Loop unrolling is a powerful optimization technique, but it can be particularly effective if the loop’s trip count (i.e., the number of iterations) is known at compile time and relatively small.
Moreover, we should check if the loop contains conditional branches or other overhead, unrolling may help reduce branching overhead by executing multiple iterations without the need for condition checks.
Also, it is important to experiment with different unrolling factors to find the optimal balance between loop overhead and instruction-level parallelism. For that, we can use profiling tools and performance metrics to evaluate the impact of loop unrolling on code performance and identify opportunities for further optimization. We can take advantage of different tools such as Intel VTune and GNU gprof that can help identify performance bottlenecks.
7. Conclusion
Whether loop unrolling is manually applied to critical sections of code or leveraged through compiler optimizations, it empowers developers to optimize their codebases and achieve tangible performance gains
In this article, we explored loop unrolling techniques, analyzed why it is important, delved into its benefits, and talked about different ways that can be applied to improve code performance.