1. Introduction
In this tutorial, we’ll learn how the Interface Segregation Principle (ISP) can vastly improve the design and functionality of software systems. ISP is a crucial concept in object-oriented programming, focusing on the structure and organization of interfaces. By adhering to this principle, we can create more flexible, maintainable, and efficient code.
2. What Are Interfaces in Computer Science
In computer science, an interface is a programming structure that outlines the methods that a class should implement. It serves as a contract, defining what behavior a class is expected to exhibit. This allows multiple classes to share a common set of behaviors without being tied to a specific implementation.
Interfaces in computer science serve as contractual blueprints, specifying the methods a class must implement. They act as a binding agreement, clearly defining the expected behavior of a class. This ingenious feature empowers various classes to inherit a uniform set of behaviors, liberating them from dependency on any particular implementation. By adopting interfaces, we pave the way for modular, adaptable, and scalable code:
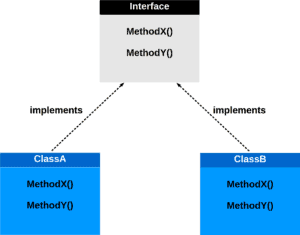
In Java, an interface is a reference type, akin to a class, capable of holding constants, method signatures, default methods, static methods, and nested types. These interfaces create a template, setting the standard for functions that any class must define. This enforces a structured approach, ensuring that any class adhering to the interface complies with the specified contract.
Interfaces also foster polymorphism, allowing objects to be manipulated uniformly regardless of their underlying types. This not only simplifies code but also enhances its flexibility and reusability. Through interfaces, Java achieves a dynamic and versatile system where classes can harmoniously cooperate, each contributing its unique functionality while adhering to a shared protocol. This concept is fundamental to object-oriented programming, enabling robust, modular, and comprehensible software development.
3. An Example of Interfaces Using Java
This code demonstrates a common scenario in object-oriented programming. We have an interface (Shape) that mandates methods for calculating area and volume, as well as for drawing the shape. While this seems logical at first, it poses a problem for 2D shapes like circles, which don’t have a volume. Despite this, they’re still required to implement a non-applicable method. This highlights the importance of designing interfaces that cater specifically to the behaviors relevant to each class:
// Example of an Interface not following ISP
interface Shape {
double calculateArea(); // Calculate area for all shapes
double calculateVolume(); // Calculate volume, not applicable for 2D shapes
void draw(); // Draw the shape
}
// Circle class implementing the Shape interface
class Circle implements Shape {
@Override
public double calculateArea() {
// Area calculation for a circle
}
@Override
public double calculateVolume() {
// This is not applicable for a circle, but we're forced to implement it.
// This goes against ISP.
}
@Override
public void draw() {
// Draw a circle
}
}
// Sphere class implementing the Shape interface
class Sphere implements Shape {
@Override
public double calculateArea() {
// Area calculation for a sphere
}
@Override
public double calculateVolume() {
// Area calculation for a sphere
}
@Override
public void draw() {
// Draw a sphere
}
}
In the above example, the Shape interface includes methods for calculating both area and volume. However, for 2D shapes like circles, calculating volume doesn’t make sense. This violates the Interface Segregation Principle (ISP) explained in the section ahead.
4. Interface Segregation Principle
The Interface Segregation Principle (ISP) is a crucial design principle in object-oriented programming that emphasizes the importance of creating lean and client-specific interfaces. It guides us away from creating bulky interfaces that encompass a multitude of methods, some of which may be irrelevant or unnecessary for certain classes. When interfaces become overly extensive, they tend to obscure the true nature of the functionalities they represent, potentially leading to code bloat and complexity.
4.1. Fat and Lean Interfaces
To grasp ISP, it’s essential to distinguish between fat and lean interfaces. A fat interface is characterized by an abundance of methods, covering a wide spectrum of functionalities:
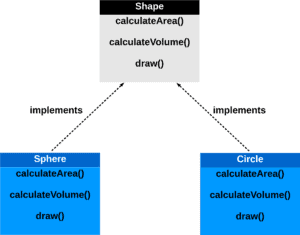
On the contrary, a lean interface is precise and exclusively contains methods that are directly pertinent to a class’s intended purpose. Lean interfaces foster a cleaner and more focused codebase by eliminating any unnecessary clutter:
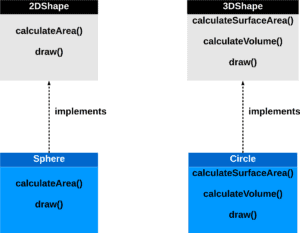
The Interface Segregation Principle advocates for the creation of interfaces that are tailored to the specific needs of the classes they serve. By shunning bulky interfaces in favor of lean and client-specific ones, developers can achieve a more focused, comprehensible, and maintainable codebase. Embracing ISP not only elevates the design and architecture of software systems but also empowers developers to navigate the complexities of modern software development with agility and precision.
4.2. The Perils of Excessive Functionality in an Interface
One of the pitfalls of incorporating excessive functionality into an interface is that it compels classes to implement methods they may not require. This not only results in code redundancy but also introduces potential points of failure or misuse. By adhering to ISP, interfaces are tailored to the precise needs of the classes, avoiding this issue altogether.
4.3. The Hazards of Interfaces Concealing Functionality
In scenarios where fat interfaces are employed, the true responsibilities and capabilities of a class can become muddled and unclear. This lack of transparency can significantly impede the understanding and maintenance of the codebase. In contrast, lean interfaces present a lucid and concise specification of a class’s capabilities, making the codebase more comprehensible and manageable.
4.4. ISP and Modular Development
ISP aligns seamlessly with the concept of modular development, which advocates for the division of a system into smaller, manageable, and independent modules. Lean interfaces promote this modularity by ensuring that each module only relies on the specific functionalities it needs. This reduces interdependencies and facilitates easier integration, testing, and debugging of individual modules.
5. Designing Lean Interfaces
Returning to our Java example, let’s redesign the interface. Instead of one monolithic interface (Shape), we’ll have multiple interfaces (TwoDimensionalShape and ThreeDimensionalShape). This adheres to the ISP by ensuring that classes only need to implement the methods that are directly relevant to their functionality.
// Example of well-designed Interfaces following ISP
interface TwoDimensionalShape {
double calculateArea(); // Calculate area for 2D shapes
void draw(); // Draw the shape
}
interface ThreeDimensionalShape {
double calculateSurfaceArea(); // Calculate surface area for 3D shapes
double calculateVolume(); // Calculate volume for 3D shapes
void draw(); // Draw the shape
}
// Circle class implementing the TwoDimensionalShape interface
class Circle implements TwoDimensionalShape {
@Override
public double calculateArea() {
// Area calculation for a circle
}
@Override
public void draw() {
// Draw a circle
}
}
// Sphere class implementing the ThreeDimensionalShape interface
class Sphere implements ThreeDimensionalShape {
@Override
public double calculateSurfaceArea() {
// Surface area calculation for a sphere
}
@Override
public double calculateVolume() {
// Area calculation for a sphere
}
@Override
public void draw() {
// Draw a sphere
}
}
6. Conclusion
In this article, we learned how the Interface Segregation Principle enhances the design of software systems by promoting lean interfaces. By avoiding fat interfaces and tailoring our interfaces to the specific needs of implementing classes, we create more focused, efficient, and maintainable code.
Adhering to ISP leads to a cleaner and more intuitive codebase, ultimately benefiting the long-term viability and scalability of our software projects.